Playwright Release 1.40 Includes Ability to Create Assertions Through Codegen Tool!
The Playwright team just released version 1.40 of Playwright, which included a long awaited feature: the ability to create assertions right from the Codegen tool! This should help lower the barrier to entry for folks new to Playwright making it easier for them to add assertions to their browser actions. One of the biggest problem I've experienced in Test Automation are UI End to End tests that don't include any assertions.
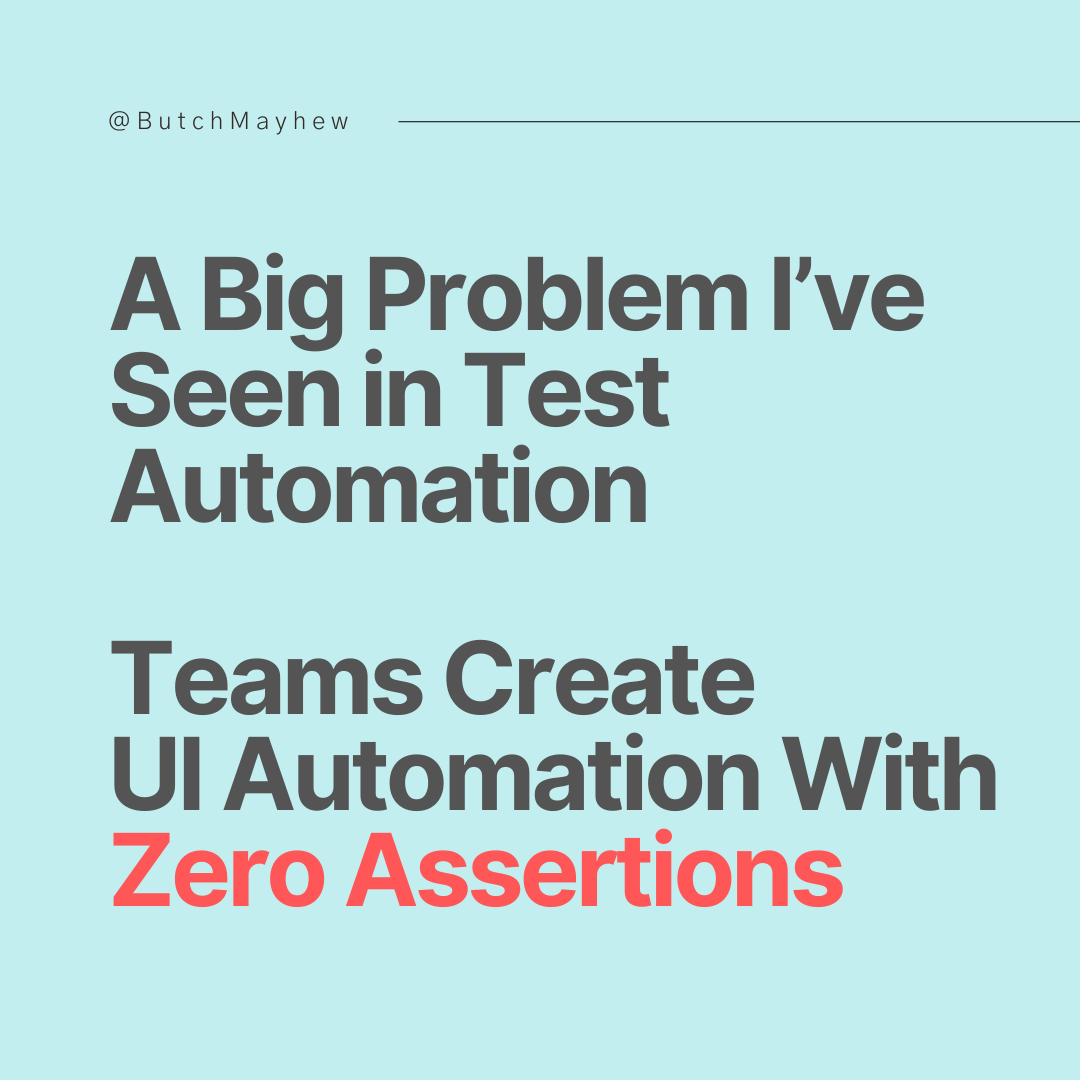
What's nice about this latest release is it give tools to quickly generate assertions right from the VS Code Playwright Extension.
A walk through of this functionality can be found below
For this specific example I wanted to visit https://practicesoftwaretesting.com/ visit a product details page and validate the Add to Cart functionality works properly. Within 2 minutes and 30 seconds you can see how quickly I was able to get a basic scenario recorded and the code for it. From here I can decide if I want to build out a page object for it if I believe there will be a lot of other similar tests, I typically go ahead and do that. For this example I'll keep the locators within the tests rather than abstracting.
import { test, expect } from '@playwright/test';
test('test', async ({ page }) => {
await page.goto('https://practicesoftwaretesting.com/');
await page.goto('https://practicesoftwaretesting.com/#/');
await page.getByTestId('nav-categories').click();
await page.getByTestId('nav-hand-tools').click();
await page.getByTestId('product-01HGNV046D6QCTCXZ8KPN3FN9A').click();
await expect(page.getByTestId('product-name')).toContainText('Claw Hammer with Shock Reduction Grip');
await expect(page.locator('app-detail')).toContainText('Hammer');
await expect(page.locator('app-detail')).toContainText('Brand name 1');
await page.getByTestId('increase-quantity').click();
await expect(page.getByTestId('quantity')).toHaveValue('2');
await page.getByTestId('add-to-cart').click();
await expect(page.getByTestId('cart-quantity')).toContainText('2');
await page.getByTestId('nav-cart').click();
await expect(page.getByRole('spinbutton')).toHaveValue('2');
await expect(page.locator('tbody')).toContainText('Claw Hammer with Shock Reduction Grip');
await page.getByTestId('proceed-1').click();
});
The recording provided the above code. In this example I am using a product under hand tools category, named Claw Hammer With Shock Reduction Grip. I went ahead and added assertions on this by validing the text of the product name locator. I also increased the quantity of items in the cart, and then validated that the new value is 2 in the different parts of the test. I then proceeded through the next page and validated that the name and values were still present and accurate.
I'll take a moment and cleanup the code, and move to a spec file that will live in the repo versus test1.spec.ts.
// tests/scenarios/addToCart.spec.ts
import { test, expect } from "@playwright/test";
test("Validate cart is updated from product details page", async ({ page }) => {
await page.goto("https://practicesoftwaretesting.com/#/");
await page.getByTestId("nav-categories").click();
await page.getByTestId("nav-hand-tools").click();
await page.getByTestId("product-01HGNV046D6QCTCXZ8KPN3FN9A").click();
await expect(page.getByTestId("product-name")).toContainText(
"Claw Hammer with Shock Reduction Grip"
);
await expect(page.locator("app-detail")).toContainText("Hammer");
await expect(page.locator("app-detail")).toContainText("Brand name 1");
await page.getByTestId("increase-quantity").click();
await expect(page.getByTestId("quantity")).toHaveValue("2");
await page.getByTestId("add-to-cart").click();
await expect(page.getByTestId("cart-quantity")).toContainText("2");
await page.getByTestId("nav-cart").click();
await expect(page.getByRole("spinbutton")).toHaveValue("2");
await expect(page.locator("tbody")).toContainText(
"Claw Hammer with Shock Reduction Grip"
);
});
I've updated the test with a proper title, and created a scenarios
directory where this check will live. One thing to note, is I am not using any sort of authentication so this check is super happy path. As I would continue to build things out I would wrap different tests within a describe block and add different scenarios. This level of testing is less around End to End testing more around ensuring that the specific functionality to add an item to a cart is working properly. So it's specifically testing javascript interactions, and does validate that the cart state is persisted after clicking into the cart. It isn't validating that someone can successfully check out.
The new functionality to be able to add assertions right from the codegen tool is a huge improvement that will lower the barrier to entry in getting started. It will definitely not solve all your problems, and if your website is not very testable (doesn't have good unique locators), the codegen tool overall is less valuable in building resilient locators for your tests.
Thanks for reading! If you found this helpful, reach out and let me know on LinkedIn or consider buying me a cup of coffee. If you want more content delivered to you in your inbox subscribe below, and be sure to leave a ❤️ to show some love.