How to quickly get realistic data with Faker?
When testing a registration process, or filling any form, it's pretty easy to add a "Jane Doe". But when you also need to add an email, an address, and do it in a different locale it becomes troublesome.
Sure you can create data sets, but why not just generate it with code ?
That's exactly what Faker does: it can generate anything from name, address, to business name. It can create realistic fake data for your testing usages.
Faker is a node library, so let's install it with npm install --save-dev @faker-js/faker
import { faker } from '@faker-js/faker/locale/en';
const randomName = faker.person.fullName(); // Rowan Nikolaus
const randomEmail = faker.internet.email(); // [email protected]
Functions to create fake data are regrouped by domain : person, company, location, date…
Integration with Playwright
Using Faker with Playwright test is easy:
import { faker } from '@faker-js/faker/locale/en';
import { expect, test } from '@playwright/test';
test.describe('Testing the application', () => {
test('should create an account with username and password', async ({
page,
}) => {
const username = faker.internet.userName();
const password = faker.internet.password();
const email = faker.internet.exampleEmail();
// Visit the webpage and create an account.
await page.goto('https://www.example.com/register');
await page.getByLabel('email').fill(email);
await page.getByLabel('username').fill(username);
await page.getByLabel('password', { exact: true }).fill(password);
await page.getByLabel('confirm password').fill(password);
await page.getByRole('button', { name: 'Register' }).click();
// Now, we try to login with these credentials.
await page.goto('https://www.example.com/login');
await page.getByLabel('email').fill(email);
await page.getByLabel('password').fill(password);
await page.getByRole('button', { name: 'Register' }).click();
// We should have logged in successfully to the dashboard page.
await expect(page).toHaveURL(/.*dashboard/);
});
});
The test is now based on a username
, password
and email
that are generated on lines 8-10.
Seeding
You will get fresh data on each test run. However, sometimes you want it to be not-so-random. For example, if you do snapshot testing, having the values changed every time will break your test.
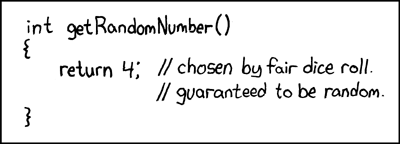
To keep your test deterministic, you'll need to seed the random generator of Faker and reset it between each test to keep everything clean.
Here is a file with a seeded test (always the same value) and a random test :
import { faker } from '@faker-js/faker/locale/en';
import { expect, test } from '@playwright/test';
// This will re-seed our faker instance after each test.
test.afterEach(() => {
faker.seed();
});
test('bear with seeded Faker', () => {
// Seed our faker instance with some static number.
faker.seed(123);
const animal = faker.animal.bear();
console.log(animal);
expect(animal).toMatchSnapshot();
});
test('random bear', () => {
const animal = faker.animal.bear();
console.log(animal);
});
Let's check the results, here with 3 runs : one for each browser.
> npx playwright test
Running 6 tests using 1 worker
✓ 1 [chromium] › seed.spec.ts:9:1 › bear with seeded Faker (75ms)
Asian black bear
✓ 2 [chromium] › seed.spec.ts:18:1 › random bear (65ms)
Giant panda
✓ 3 [firefox] › seed.spec.ts:9:1 › bear with seeded Faker (62ms)
Asian black bear
✓ 4 [firefox] › seed.spec.ts:18:1 › random bear (29ms)
American black bear
✓ 5 [webkit] › seed.spec.ts:9:1 › bear with seeded Faker (58ms)
Asian black bear
✓ 6 [webkit] › seed.spec.ts:18:1 › random bear (27ms)
Spectacled bear
6 passed (2.5s)
As you can see, the bear with seeded Faker
test is based on snapshot. It passes without problem, and it will on every consequent runs. The console output is always Asian black bear
The random bear
console output is different on each test run. This is because after each test, we reset the Faker seed.
See more details in Faker's documentation section on Reproducible results
Wrap up
Faker is a handy library to generate fake, but realistic, data. We've seen its basic usage, and how to add it to our tests, with and without randomness on each run. Turns out Faker is easy to use with Playwright Test !
Hi! I’m Jean-François, and I’m a Front-end Tech Lead. I help teams on their journey to more agility and technical excellence. I’m interested in Software Craft and testing.
Find me at https://jfgreffier.com