How do I check the value inside an input field with Playwright?
Between all the assertions you can make on a page while on a page in playwright, every once in a while I find I want to validate that text is already pre-populated in an input field to validate my system is behaving properly.
In this case the assertions aren't super straight forward, a typical approach would be to find the element on the page, and look for .text or .innerText, the problem with input fields is they typically aren't apart of the dom.
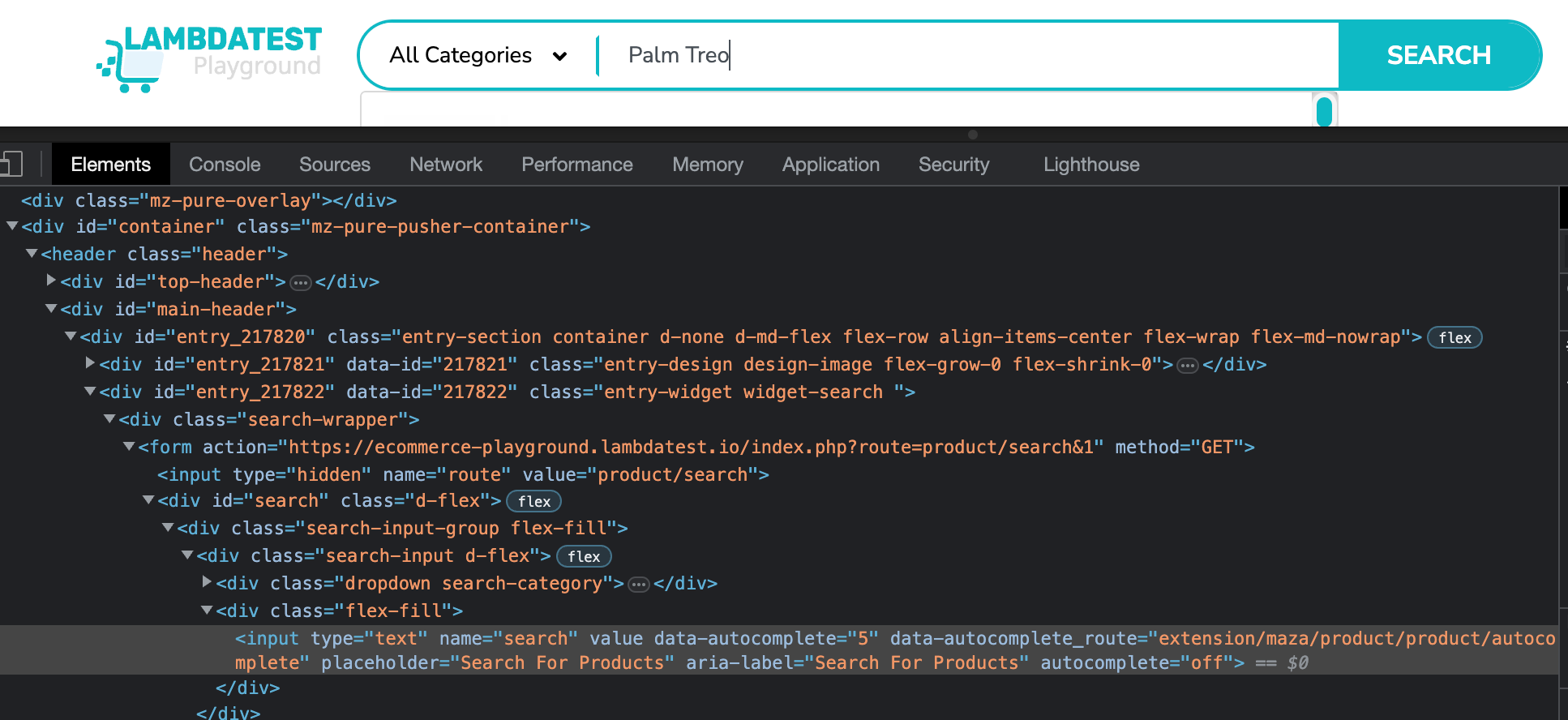
But thankfully Playwright has a way to handle this!
For this example I will be using the test site https://ecommerce-playground.lambdatest.io/. This site is a fake storefront that we can write automation against. My test scenarios will be:
- Use the search box to search for a product and click search
- Validate that he product name I searched for is still in search box
- Click onto a product details page and validate product name is still in search box
- Click on add to cart and validate product name is still in search box
- Click checkout, and validate the product name is no longer in the search box
Whether the last point is how it should function is not something I am questions at the moment, but could be brought as a question, it is how the system operates at this time, so it makes sense to add an automated check for it. If it changes our automation will let us know it changed.
Using .toHaveValue() assertion method
Using this method is delightful. This method can be used on any locator and is known as a LocatorAssertion. These are first class citizens and do require you to use await
with your expect . The below example shows off how to use this function.
test("Validate product search input", async ({ page }) => {
await page.goto("https://ecommerce-playground.lambdatest.io/");
const productName = "Palm Treo Pro";
const productSearch = page.getByRole("textbox", {
name: "Search For Products",
});
const searchButton = page.getByRole("button", { name: "Search" });
await productSearch.fill(productName);
await test.step("persists after running a search", async () => {
await searchButton.click();
await expect(productSearch).toHaveValue(productName);
});
});
The official Playwright docs are linked below
Using the clipboard to make the assertion
A 2nd way to accomplish this without using the toHaveValue(), which could come in hand for tricky scenarios is to click into the input box, select the input and copy it to the clipboard. Then use JavaScript to get the contents of the clipbard, and assert on that. The code for this example is below.
import { test, expect } from "@playwright/test";
test("Validate product search input", async ({ page }) => {
await page.goto("https://ecommerce-playground.lambdatest.io/");
const productName = "Palm Treo Pro";
const productSearch = page.getByRole("textbox", {
name: "Search For Products",
});
const searchButton = page.getByRole("button", { name: "Search" });
await productSearch.fill(productName);
await test.step("persist after running a search alternate method", async () => {
await productSearch.click();
let userAgentInfo = await page.evaluate(() => navigator.userAgent);
if (userAgentInfo.includes("Mac OS")) {
await page.keyboard.press("Meta+A");
await page.keyboard.press("Meta+C");
} else {
await page.keyboard.press("Control+A");
await page.keyboard.press("Control+C");
}
let clipboardText = await page.evaluate("navigator.clipboard.readText()");
expect(clipboardText).toBe(productName);
});
});
In this example we first evaluate the userAgent of the browser to know what keyboard modifier we need to press to copy and paste, if Mac OS we will use the Meta/Command key, if Windows or Linux we will use the Control modifier key. We first click into the productSearch locator so our cursor is in the input field, then we press the shortcut for Select and then shortcut for Copy. The next step of the code is getting the clipboardText.
use: { permissions: ["clipboard-read"] }
The code then sets clipboardText to the clipboard text, and then makes an assertion to ensure that the text in the clipboard matches the original productName variable.
Ideally you're not having to do this, but it is possible :) The full spec can be found below, notice I added my different scenarios using test.steps which makes it nice to debug.
import { test, expect } from "@playwright/test";
test("Validate product search input", async ({ page }) => {
await page.goto("https://ecommerce-playground.lambdatest.io/");
const productName = "Palm Treo Pro";
const productSearch = page.getByRole("textbox", {
name: "Search For Products",
});
const searchButton = page.getByRole("button", { name: "Search" });
await productSearch.fill(productName);
await test.step("persists after running a search", async () => {
await searchButton.click();
await expect(productSearch).toHaveValue(productName);
});
await test.step("persist after running a search alternate method", async () => {
await productSearch.click();
let userAgentInfo = await page.evaluate(() => navigator.userAgent);
if (userAgentInfo.includes("Mac OS")) {
await page.keyboard.press("Meta+A");
await page.keyboard.press("Meta+C");
} else {
await page.keyboard.press("Control+A");
await page.keyboard.press("Control+C");
}
let clipboardText = await page.evaluate("navigator.clipboard.readText()");
expect(clipboardText).toBe(productName);
});
await test.step("persists after navigating to the product page", async () => {
await page.getByRole("link", { name: productName }).nth(1).click();
await expect(productSearch).toHaveValue(productName);
});
await test.step("persists after adding product to cart", async () => {
await page.getByRole("button", { name: "Add to Cart" }).click();
await expect(productSearch).toHaveValue(productName);
});
await test.step("does not persists when visiting checkout", async () => {
await page.getByRole("link", { name: "Checkout " }).click();
await expect(productSearch).not.toHaveValue(productName);
});
});
The full code additions can be found at the pull request link below.
Thanks for reading! If you found this helpful, reach out and let me know on LinkedIn or consider buying me a cup of coffee. If you want more content delivered to you in your inbox subscribe below.