What the Hex, or How I Check Colors With Playwright

Don't worry; it's not a Matrix situation where you have to choose between red and blue. I'm a visual person, and it's easier for me to explain things with diagrams, pictures, or, better yet, my facial expressions. Since the internet isn't ready for my facial expressions, let's use pictures here.
Playwright does an amazing job when running my sign in check. It finds the "Sign in" button using our data test id and clicks on it. However, the application I am working on allows end-users customize colors, meaning an admin can change the color of buttons and backgrounds. This is not something I want to spend every release testing so I had to put playwright abilities to test. Can I easily check the color of the button using Playwright out of the box? Or will I end up evaluating JavaScript in the end?
Turns out, you can check the color of the button. All you have to do is use .toHaveCSS()
(link to official docs)
Of course, I'm not going to leave you here with just the link above and wish you a good day. Let's write an assertion for the button together.
It all starts with inspecting the element you want to check the color for. In our case, it's the 'Sign in' button, and here's how it looks in DevTools:
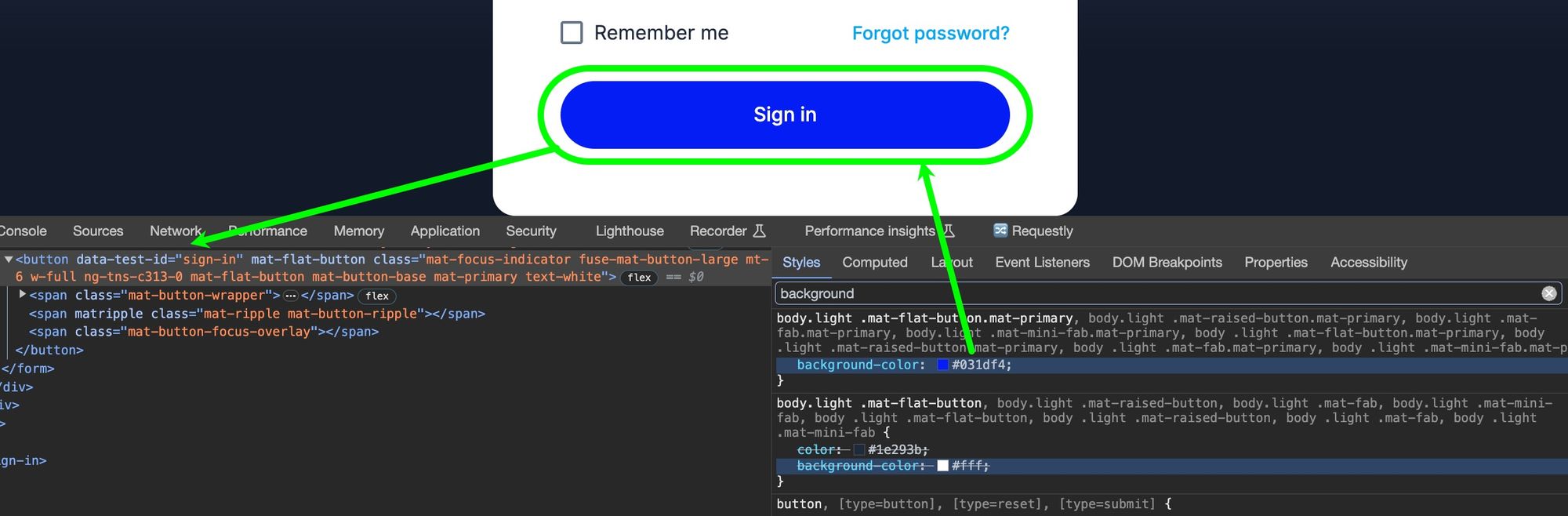
When you click on the element, you'll find a lot of CSS properties. Here's a helpful hack: use the search box in the styles tab on the right hand side and type 'background' or 'color' to filter out the specific properties you need.
As you can see in this example, the background color is not just blue, but "#031df4". This is the hexadecimal code for that specific color.
* If you are feeling a little bit lost right now, you can simply Google "hex color codes explained" and come back with better understanding.
We have everything we need per documentation:
- locator (sign-in button)
- CSS property name (
background-color
) - CSS property value (
#031df4
).
Let's write the check and run it:
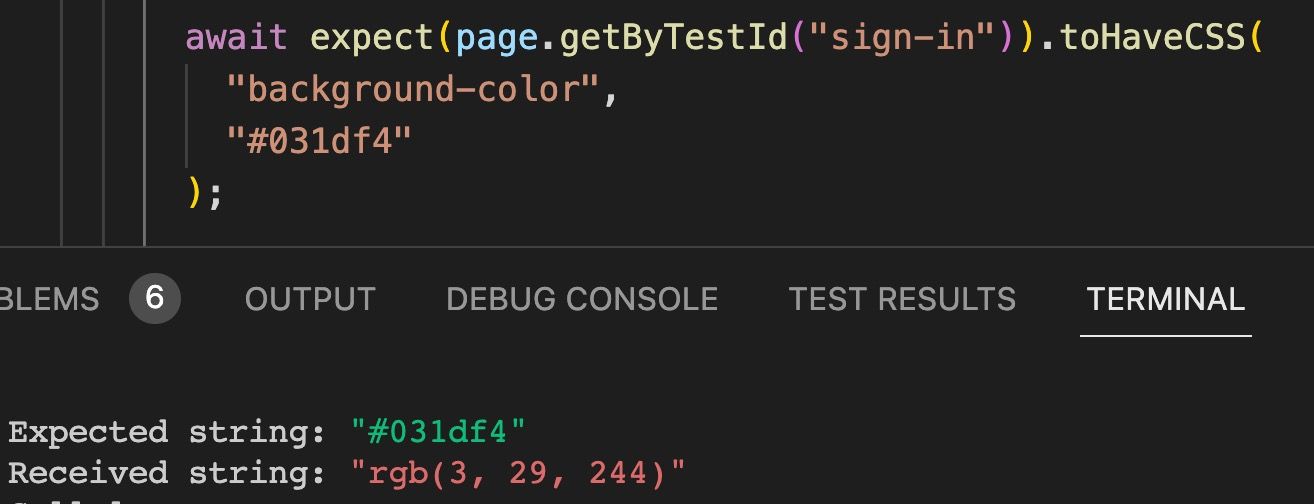
It fails because .toHaveCSS()
expects you to pass RGB colors for the "background-color" in the specific format.
* If you are feeling a little bit lost right now, you can simply Google "RGB color codes explained" and, maybe, "RGB vs hex" for a better understanding.
You'll need to convert the hex color code to an RGB color code. After a quick Google search, I found a straightforward solution that doesn't require the use of a library:
export function convertHexToRGB(hex) {
// Remove the '#' if it's included in the input
hex = hex.replace(/^#/, '');
// Parse the hex values into separate R, G, and B values
const red = parseInt(hex.substring(0, 2), 16);
const green = parseInt(hex.substring(2, 4), 16);
const blue = parseInt(hex.substring(4, 6), 16);
// Return the RGB values in an object
return {
red: red,
green: green,
blue: blue,
};
}
I saved it to my ./lib
folder and then imported to the spec file:
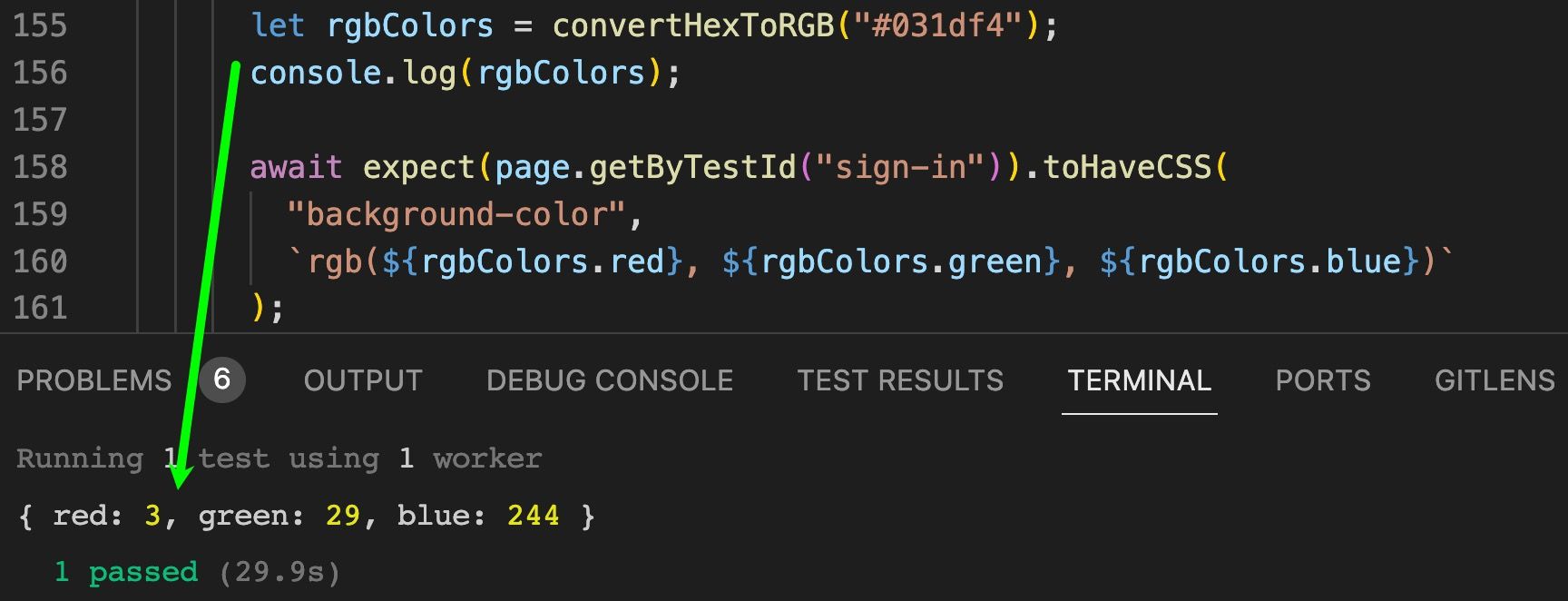
The test has passed! Now, I have a check in place to ensure that the button's color is exactly what I expect it to be. I've also added checks for disabled buttons. This way, if the code for disabled buttons ever changes, I'll be the first to know. (Note: disabled buttons in my application didn't use the "background-color" property but the "color" property, so always be diligent and check properties manually first.)
If you have multiple .toHaveCSS()
assertions in a spec, it's a good practice to abstract them into your "./lib" folder (or wherever you keep your fixtures) to improve code readability.
export async function checkColor(element, cssProperty, rgbColors) {
await expect(element).toHaveCSS(cssProperty, `rgb(${rgbColors.red}, ${rgbColors.green}, ${rgbColors.blue})`);
}
Finally, here's an example of how color checks can look in a spec file with a page object pattern, abstracted convertHexToRGB()
, and checkColor()
:

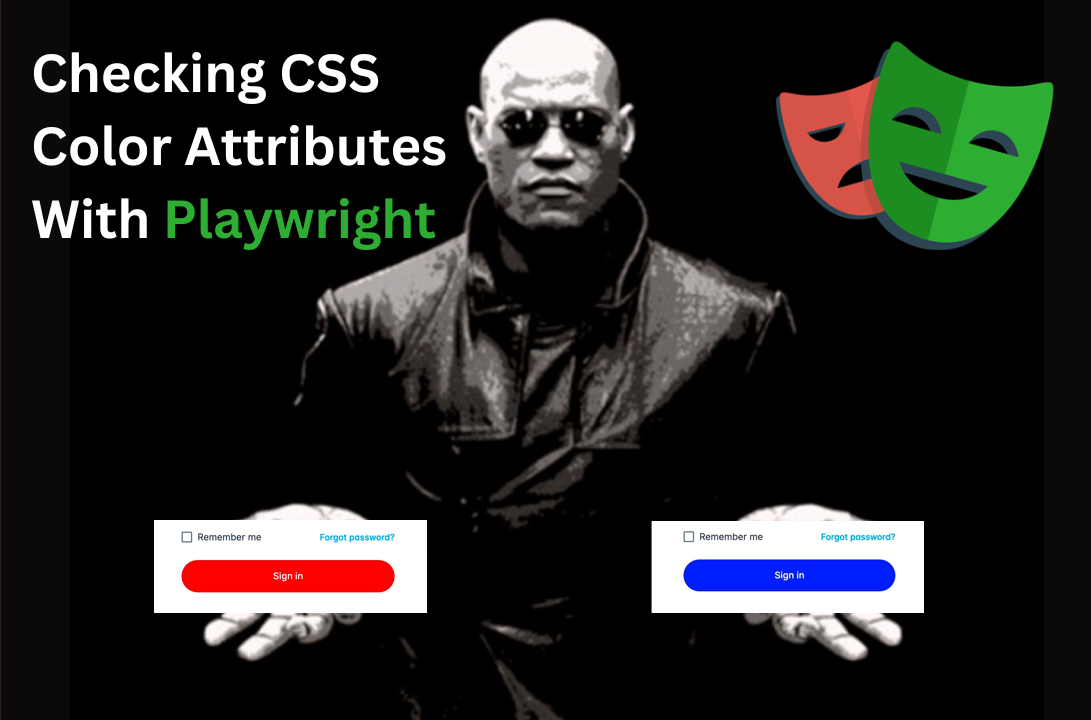
I hope you find this useful, and if you did, please ❤️ and subscribe below to receive more useful tips. If you want to reach out to me personally, feel free to connect or message on LinkedIn.